Using Firebase Crashlytics
Notice
This page is archived and might not reflect the latest version of the FlutterFire plugins. You can find the latest information on firebase.google.com:
#
Getting startedEnable Firebase Crashlytics in the Firebase console.
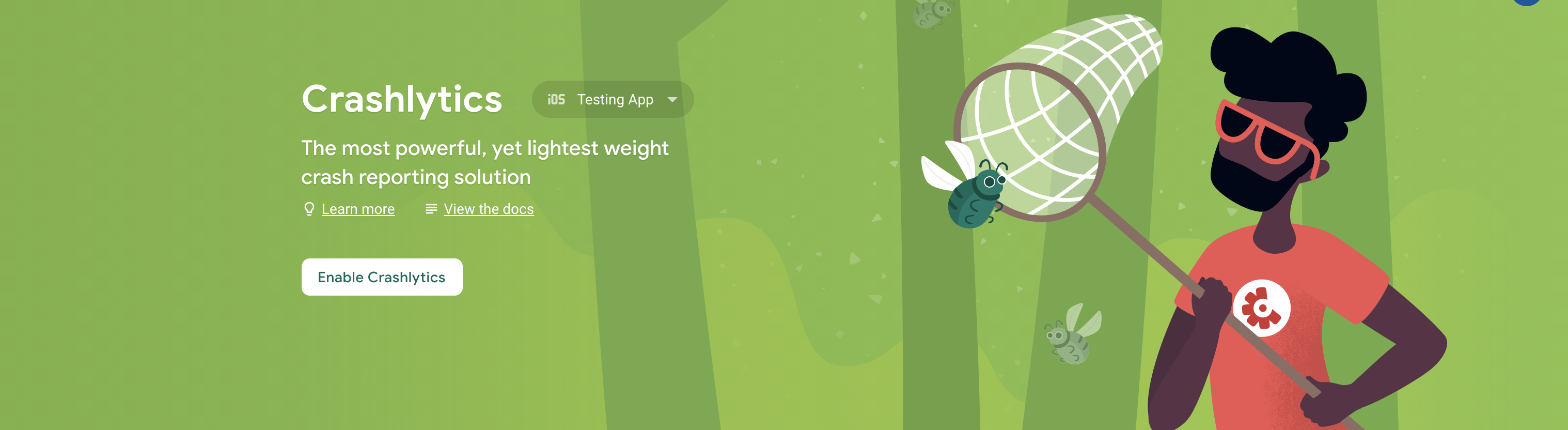
Until an error has been reported, you will see this screen.
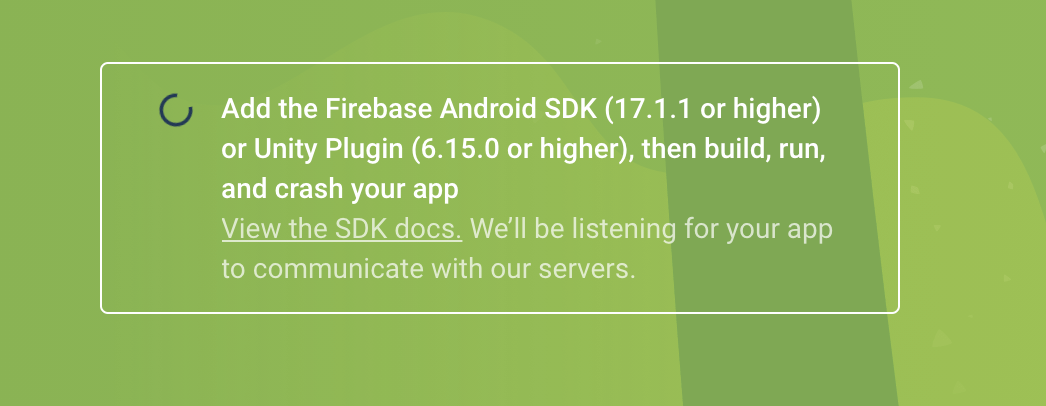
To start using Firebase Crashlytics within your project, import it at the top of your project files:
Run an example, such as...
This will crash the currently running application. You will then need to manually re-run your application on your emulator for Crashlytics to submit the crash report to the Firebase Console.
#
Sending reports to CrashlyticsTo send report data to Crashlytics, the application must be restarted. Crashlytics automatically sends any crash reports to Firebase the next time the application is launched.
#
Toggle Crashlytics collectionCall the setCrashlyticsCollectionEnabled
method to toggle Crashlytics collection status.
For example to ensure it is disabled when your app is in debug mode you can do the following:
You can additionally read the current collection enabled status:
#
Forcing a crashYou don't have to wait for a crash to know that Crashlytics is working. To force a crash, call the crash
method:
Your app should exit immediately after calling this method. After opening your app again after the crash Firebase Crashlytics will upload the crash report to the Firebase Console.
The error will be shown on the Firebase Crashlytics dashboard as an instance of FirebaseCrashlyticsTestCrash
, with a message of
This is a test crash caused by calling .crash() in Dart.
#
Crash types#
Fatal crashIf you would like to record a fatal error, you may pass in a fatal
argument as true
.
The crash report will appear in your Crashlytics dashboard with the event type Crash
, the event summary stack trace will also be referenced as a Fatal Exception
.
#
Non-Fatal crashBy default non-fatal errors are recorded. The crash report will appear in your Crashlytics dashboard with the event type Non-fatal
, the event summary stack trace will also be referenced as a Non-fatal Exception
.
#
Add custom keysTo associate key/value pairs with your crash reports, you can use the setCustomKey
method
This accepts a maximum of 64 key/value pairs. New keys beyond that limit are ignored. Keys or values that exceed 1024 characters are truncated.
#
Add custom log messagesTo add custom Crashlytics log messages to your app, use the log
method
#
Set user identifiersTo add user IDs to your reports, assign each user with a unique ID. This can be an ID number, token or hashed value:
To reset a user ID (e.g. when a user logs out), set the user ID to an empty string.
#
Handling uncaught errorsBy overriding FlutterError.onError
with FirebaseCrashlytics.instance.recordFlutterError
, it will automatically
catch all errors that are thrown within the Flutter framework.
#
Zoned ErrorsNot all errors are caught by Flutter. Sometimes, errors are instead caught by Zones.
A common case were FlutterError
would not be enough is when an exception happen
inside the onPressed
of a button:
To catch such errors, you can use runZonedGuarded
like do:
Note that you must call WidgetsFlutterBinding.ensureInitialized()
inside runZonedGuarded
. Error handling wouldn’t work if WidgetsFlutterBinding.ensureInitialized()
was called from the outside.
#
Errors outside of FlutterTo catch errors that happen outside of the Flutter context, install an error listener on the current Isolate:
#
Enable opt-in reportingBy default, Crashlytics will automatically collect crash reports for all your app's users. To give users more control over the data they send, you can enable opt-in reporting by disabling automatic collection and initializing Crashlytics only for selected users:
- Turn off automatic collection natively:
a. Android
In the application
block of your AndroidManifest.xml
file, add a meta-data
tags to turn off automatic collection:
b. iOS
Add a new key to your Info.plist
file.
- Key:
FirebaseCrashlyticsCollectionEnabled
- Value:
false
- Enable collection for select users by calling the Crashlytics data collection override at runtime. To opt out of automatic
crash reporting, pass
false
as the override value. When set tofalse
, the new value does not apply until the next run of the app.