Theming
Notice
This page is archived and might not reflect the latest version of the FlutterFire plugins. You can find the latest information on GitHub:
https://github.com/firebase/flutterfire/blob/master/packages/flutterfire_ui/doc/auth/theming.md
FlutterFire UI widgets are built on top of Material and Cupertino design patterns provided by Flutter.
To provide consistency across your application, the FlutterFire UI widgets depend on the ThemeData
or CupertinoThemeData
instances provided to your MaterialApp
or CupertinoApp
widget.
For example, the SignInScreen
widget with an email provider, wrapped in a MaterialApp
will use the following widgets:
This will render a screen with the default Material style widgets:
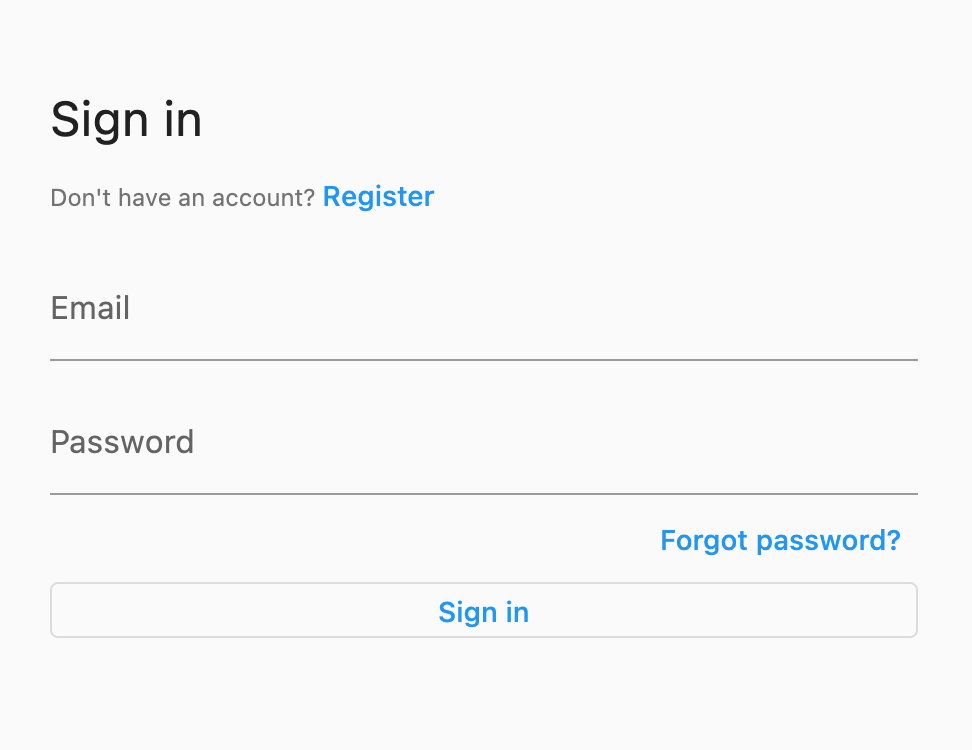
To update these styles, we can override the ThemeData
provided to the MaterialApp
. For example, to apply a border to the input fields,
we can override the InputDecorationTheme
:
The UI widgets will respect the updated theme data, and the UI will be reflected to match:
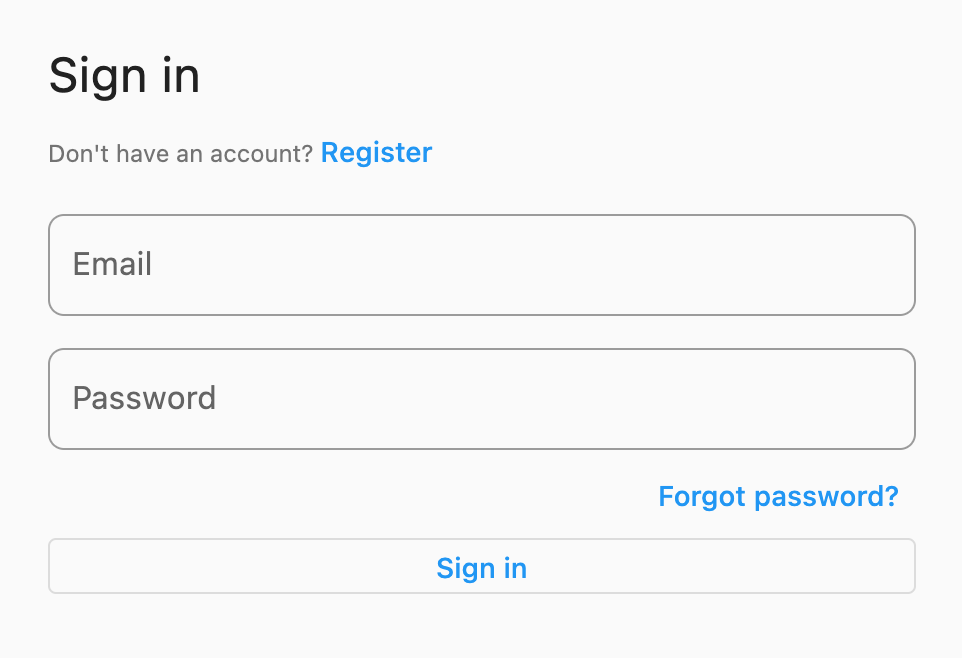
Furthermore, we can customize the button used in the UI by overriding the OutlinedButtonThemeData
:
The button will now respect the updated theme data and display a styled button instead:
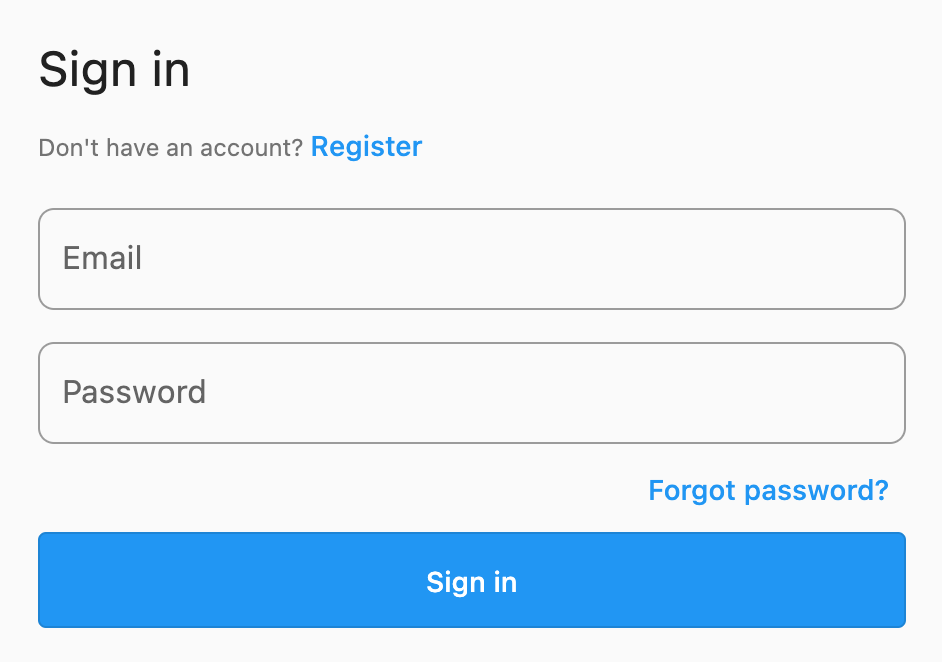